Custom Post Type Snippets to make you smile
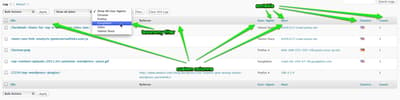
So it’s friday, I’ve been coding all day and I thought I’d share some of the cool snippets I’ve come across and/or developed today. I’ve mostly been working with Custom Post Types and Taxonomies, so let me share some of that goodness. Let’s geek out in a bit, but first let me show you why this is cool, be sure to click the image, so you can see which functionality I’ve added to the otherwise boring custom posts overview screen:
Add columns to the overview page for a Custom Post Type
So you’ll want to add some columns to your post type’s overview page, or remove some. Don’t forget to replace <CPT> with your own custom post type in all these examples:
[code lang=”php”]// Change the columns for the edit CPT screen
function change_columns( $cols ) {
$cols = array(
‘cb’ => ‘<input type="checkbox" />’,
‘url’ => __( ‘URL’, ‘trans’ ),
‘referrer’ => __( ‘Referrer’, ‘trans’ ),
‘host’ => __( ‘Host’, ‘trans’ ),
);
return $cols;
}
add_filter( "manage_<CPT>_posts_columns", "change_columns" );[/code]
Be sure to always leave the cb
column in there or your mass edit / delete functionality will not work.
Give these new columns some content
Now let’s fill these new columns with some content from the custom post type:
[code lang=”php”]function custom_columns( $column, $post_id ) {
switch ( $column ) {
case "url":
$url = get_post_meta( $post_id, ‘url’, true);
echo ‘<a href="’ . $url . ‘">’ . $url. ‘</a>’;
break;
case "referrer":
$refer = get_post_meta( $post_id, ‘referrer’, true);
echo ‘<a href="’ . $refer . ‘">’ . $refer. ‘</a>’;
break;
case "host":
echo get_post_meta( $post_id, ‘host’, true);
break;
}
}
add_action( "manage_posts_custom_column", "custom_columns", 10, 2 );[/code]
Make these new columns sortable
Now this extra info is cool, I bet you want to sort by it, that’s as simple as this:
[code lang=”php”]// Make these columns sortable
function sortable_columns() {
return array(
‘url’ => ‘url’,
‘referrer’ => ‘referrer’,
‘host’ => ‘host’
);
}
add_filter( "manage_edit-<CPT>_sortable_columns", "sortable_columns" );[/code]
Filter Custom Posts by Custom Taxonomy
Ok so far this is all fairly simple. Now let’s go a bit more advanced. Let’s say you have a custom taxonomy attached to that custom post type and you want to show a filter for that custom taxonomy on the custom post types overview page, just like you have a categories drop down on the posts overview page. This code was taken (though slightly modified) from this thread.
Let’s first add that dropdown / select box to the interface:
[code lang=”php”]// Filter the request to just give posts for the given taxonomy, if applicable.
function taxonomy_filter_restrict_manage_posts() {
global $typenow;
// If you only want this to work for your specific post type,
// check for that $type here and then return.
// This function, if unmodified, will add the dropdown for each
// post type / taxonomy combination.
$post_types = get_post_types( array( ‘_builtin’ => false ) );
if ( in_array( $typenow, $post_types ) ) {
$filters = get_object_taxonomies( $typenow );
foreach ( $filters as $tax_slug ) {
$tax_obj = get_taxonomy( $tax_slug );
wp_dropdown_categories( array(
‘show_option_all’ => __(‘Show All ‘.$tax_obj->label ),
‘taxonomy’ => $tax_slug,
‘name’ => $tax_obj->name,
‘orderby’ => ‘name’,
‘selected’ => $_GET[$tax_slug],
‘hierarchical’ => $tax_obj->hierarchical,
‘show_count’ => false,
‘hide_empty’ => true
) );
}
}
}
add_action( ‘restrict_manage_posts’, ‘taxonomy_filter_restrict_manage_posts’ );[/code]
And then, we add a filter to the query so the dropdown will actually work:
[code lang=”php”]function taxonomy_filter_post_type_request( $query ) {
global $pagenow, $typenow;
if ( ‘edit.php’ == $pagenow ) {
$filters = get_object_taxonomies( $typenow );
foreach ( $filters as $tax_slug ) {
$var = &$query->query_vars[$tax_slug];
if ( isset( $var ) ) {
$term = get_term_by( ‘id’, $var, $tax_slug );
$var = $term->slug;
}
}
}
}
add_filter( ‘parse_query’, ‘taxonomy_filter_post_type_request’ );[/code]
Note that for these last two snippets to work, query_var
must have been set to true when registering the custom taxonomy, otherwise this’ll never work.
Bonus: Add Custom Post Type to feed
This one came courtesy of Remkus de Vries a while back and was helpful today, adding a custom post type to your site’s main feed, don’t forget to replace <CPT> with your own custom post type:
[code lang=”php”]// Add a Custom Post Type to a feed
function add_cpt_to_feed( $qv ) {
if ( isset($qv[‘feed’]) && !isset($qv[‘post_type’]) )
$qv[‘post_type’] = array(‘post’, ‘<CPT>’);
return $qv;
}
add_filter( ‘request’, ‘add_cpt_to_feed’ );[/code]
Coming up next!
-
Pride Amsterdam 2025
August 02, 2025 Team Yoast is at Attending Pride Amsterdam 2025! Click through to see who will be there, what we will do, and more! See where you can find us next » -
Webinar: How to start with SEO (July 14, 2025)
14 July 2025 Learn how to start your SEO journey the right way with our free webinar. Get practical tips and answers to all your questions in the live Q&A! All Yoast SEO webinars »
One thing that is confusing me is where these snippets go. Are they all in the functions.php file or in multiple files? If in multiple files maybe you can update your post to tell which file each snippet goes into. Thanks!
This is a wonderful tutorial, I am using it on a site that I am working on now. One thing that is not intuitive to me is why you can only fire the functions to “Filter Custom Posts by Custom Taxonomy” once, even if you create more than one custom post type. If they run more than once, none of them work. Oh, well, great stuff.
Where is the Order field in Custom Post Types? Is this something that has to be added when registering the CPT?
Just answered my own question. Added the Page Attributes to the Supports argument when I Register Post Types.
nice find, great snippets, bookmarked for future reference :)
Excellent information, thanks for the snippet/tutorial!
Thanks Joost, this is stuff I can actually use on some projects I’m working on right now.. Thanks for sharing!
Sander
Another tip to add our CPT in the right now widget on the dashboard
function cpt_in_right_now() {
$num_cpt = wp_count_posts( 'CPT' );
echo '';
$num = number_format_i18n( $num_cpt->publish );
$text = _n( 'CPT-NAME-SINGULAR', 'CPT-NAME-PLURAL', $num_cpt->publish );
if ( current_user_can( 'edit_post' ) ) { // or edit_CPT if defined
$num = "$num";
$text = "$text";
};
echo '' . $num . '';
echo '' . $text . '';
echo '';
}
add_action('right_now_content_table_end','cpt_in_right_now');
enjoy
Really helpfull, but some remarks :
first snippet : use
manage_edit-CPT_columns
second snippet : use
manage_CPT_posts_custom_column
or
global $post;
etc..if ($post->post_type == "CPT") :
switch (
Good stuff here, I’d done some custom column stuff for my CPTs before, but this is icing on the cake so to speak!
I agree with the others, great work as always.
You actually just given me a great idea, thanks :-)
If you intend to build a plugin …. let us know :)
Man, awesome info you put out on your site, I put “Quix” in my tool bar two days ago, mind boggling what you can do with that tool. Installed Robots Meta on 3 sites we run, 1st site saw a 1000% increase in long tail keywords in the first month, second site saw a 200% increase, 3rd site 100% increase. Consider me a huge fan of your work :) Commenting so I can subscribe to your newsletter.
Great article! I’ve been toying around with custom post types a lot lately, adding / sorting columns isn’t something I’ve considered before – thanks!
That’s not a snippet… It’s a tutorial ! Thanks for sharing !
This is a tutorial with great snippets. Joost just knows that most of us just end up copy and pasting anyway.
This is essential for plugin developers to learn. I have implemented columns and filters on my Promotion Slider plugin, but never really got around to making the columns sortable. Thanks again for a great post!
If you use any Joost’s code do not forget to give him some credits :)
Micah Wood, just kidding :)
Ha ha :) You do have to be careful though, Joost’s code is so well written, that if you look to see how he did something, you can’t help but be tempted just to copy it! But hey, I have no problem giving credit where credit is due…
Yes Micah, especially since these credits add value to your plugin. I am not a programmer, but I peek the code of plugins that I use and I like it when I see mentions with credits given to reputable developers … gives me confidence in the product overall ;)