Lazy programmers are good programmers
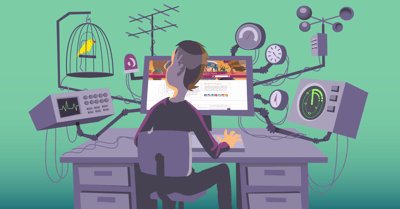
Being lazy is often not a word you want to be described as. If I call you lazy, you would object, right? But lazy is not that bad, and being a lazy programmer is certainly not a bad thing.
Bill Gates allegedly said: “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Whether Bill Gates actually said it, I do like this quote, especially when we’re talking about developers. Let me give you a couple of reasons why I think lazy programmers are good programmers.
A lazy programmer
…will not reinvent the wheel
This will save you time and can result in a cleaner and simpler codebase.
When presented with an issue or a bug, first investigate if someone else had a similar problem before you. You can use that solution to solve your problem. If you don’t repeat yourself, you might not even extend your codebase with much more lines than strictly needed.
Sometimes such a ready-made solution will not be available in your current codebase. Don’t give up, you can probably find other solutions online. For WordPress for example, there are countless plugins available that can solve a problem for you. See also this blog from Andy.
…works DRY and KISS
Your codebase will be easier to understand and maintain.
Two good principles to remind yourself of are DRY and KISS. DRY stands for Don’t Repeat Yourself. A lazy programmer will usually like this approach of writing code. For example in CSS, try to use some generic classes. Say you want to create a nice, yellow button. You could use this CSS.
.yellow-button { padding: 8px 10px 10px 10px; font-size: 1rem; border: 0; color: #000; border-radius: 4px; box-shadow: 0 2px 4px 0 rgba(0,0,0,.2),inset 0 -4px 0 rgba(0,0,0,.2); background-color: #fec228; }
And then you decide you also want a purple button.
.purple-button { padding: 8px 10px 10px 10px; font-size: 1rem; border: 0; color: #000; border-radius: 4px; box-shadow: 0 2px 4px 0 rgba(0,0,0,.2), inset 0 -4px 0 rgba(0,0,0,.2); background-color: #a4286a; }
That’s a lot of extra lines for adding just one purple button. The lazy way, and DRY way, to do this:
.button { padding: 8px 10px 10px 10px; font-size: 1rem; border: 0; color: #000; border-radius: 4px; box-shadow: 0 2px 4px 0 rgba(0,0,0,.2),inset 0 -4px 0 rgba(0,0,0,.2); background-color: #fec228; } .button.purple { background-color: #a4286a; }
KISS stands for “Keep it simple, stupid”. An easy way to keep your code simple, is to let every part of your code do only one thing. If you have a JavaScript function that’s doing two things: split it up and create two functions. This makes it easy to reuse your code, so you can be lazy the next time you might need it. This also works for files, especially if you’re using a framework like React. Make sure that every file is responsible for only one function or one part of the functionality of your project.
CSS has multiple shorthands you can use. A couple of these really come in handy when writing CSS, but there are also some you better avoid. If you want to use a specific font family and font size, you can use the shorthand:
body { font: normal small-caps bold 15px/150% Roboto, Helvetica, sans-serif; }
But this makes things overly complicated and it’s not easy to read or understand. You can ignore the values you don’t want to set, but you can end up overwriting defaults you don’t want to overwrite. It’s much simpler to just use:
body { font-family: Roboto, Helvetica, sans-serif; font-size: 13px; }
This also goes for the background shorthand.
body { background: red url(image.jpg) top center / cover no-repeat fixed padding-box content-box; }
If you want to only set a background-image, it’s better to use the property itself.
body { background-image: url(image.jpg); }
If you adhere to these principles, your code will be easy to read, easy to maintain and be as small as can be.
…automates tasks
Save time and minimize your chance of bugs.
If a programmer has to do the same job twice, they will. If a lazy programmer has to do the same job twice, they write a program that will do it for them.
Sometimes writing code to automate a task will require more work than the actual task, but in the end, it can certainly save you time. As a developer, there are a lot of tasks you can automate. You can, for example, automate processes to watch and build your code and even deploy your code automatically. Tests are also a good task you can automate.
Automating these tasks will definitely benefit you and your code. Especially when you write tests or use a service like Travis, you can minimize the chance of putting bugs or broken functionality live.
…documents everything
Helps you and others understand what you did.
This may seem ambiguous at first because writing documentation means writing more. But a lazy programmer will write as much documentation as possible. This ensures that you don’t have to explain your code, at least not more than once. And it also helps you in the future. Sometimes the code you’re writing one day, won’t make sense the other day.
Be lazy
As said, being a lazy programmer is not a bad thing. If you try to work DRY and KISS, automate your tasks and write documentation, your code will definitely benefit from your laziness. Do you want to be a lazier programmer? This should be a good starting point for you. And if someone calls you a lazy programmer, you can take pride in it. It means you can find easy ways to do difficult things.
Coming up next!
-
WordSesh 2024
July 30 - 31, 2024 Team Yoast is at Sponsoring WordSesh 2024! Click through to see who will be there, what we will do, and more! See where you can find us next » -
The SEO update by Yoast - July 2024 Edition
30 July 2024 Get expert analysis on the latest SEO news developments with Carolyn Shelby and Alex Moss. Join our upcoming update! 📺️ All Yoast SEO webinars »